Add new plugin
- dialog : It is a plugin in the form of a dialog
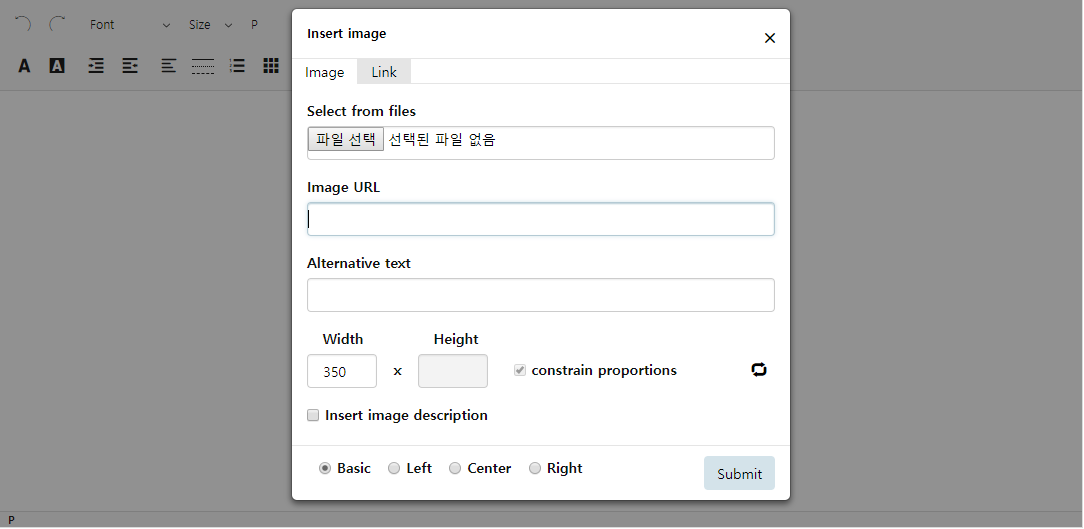
- submenu : It is a plugin in the form of a submenu
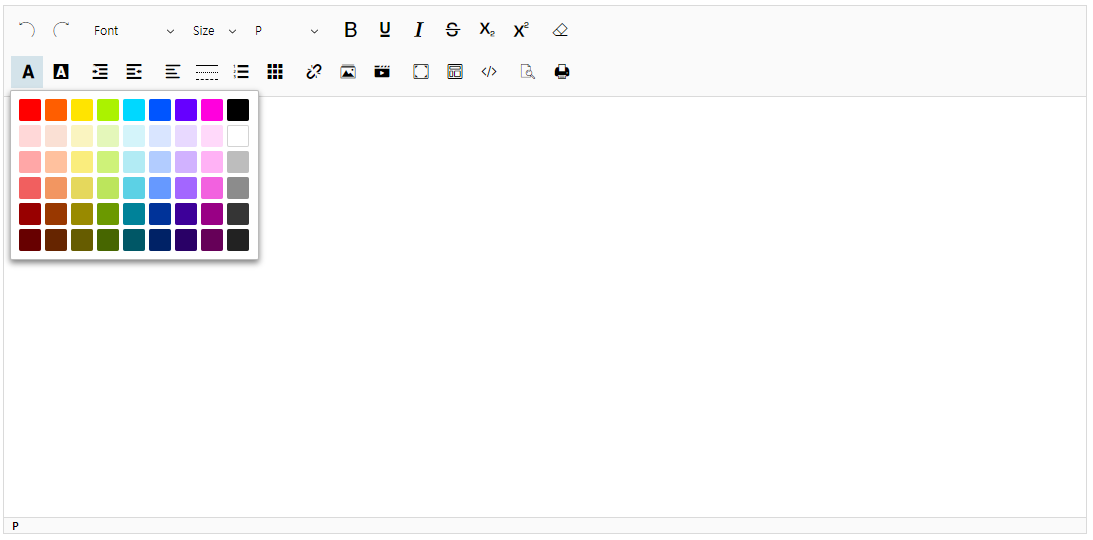
// ex) A submenu plugin that appends the contents of the input element to the editor
export default {
// plugin name (Required)
name: 'custom_plugin_submenu',
// add function - It is called only once when the plugin is first run.
// This function generates HTML to append and register the event.
// arguments - (core : core object, targetElement : clicked button element)
add: function (core, targetElement) {
// Registering a namespace for caching as a plugin name in the context object
const context = core.context;
context.custom = {
textElement: null
};
// Generate submenu HTML
// Always bind "core" when calling a plugin function
let listDiv = this.setSubmenu.call(core);
// Input tag caching
context.custom.textElement = listDiv.getElementsByTagName('INPUT')[0];
// You must bind "core" object when registering an event.
/** add event listeners */
listDiv.getElementsByTagName('BUTTON')[0].addEventListener('click', this.onClick.bind(core));
/** append html */
targetElement.parentNode.appendChild(listDiv);
},
setSubmenu: function () {
const lang = this.lang;
const listDiv = this.util.createElement('DIV');
listDiv.className = 'ke-list-layer';
listDiv.innerHTML = '' +
'<div class="ke-list-inner ke-list-align">' +
' <ul class="ke-list-basic">' +
' <li><input class="ke-input-form" type="text" style="width: 100%; border: 1px solid #CCC;" /></li>' +
' <li><button type="button" class="ke-btn ke-tooltip">' +
' <span>OK</span>' +
' <span class="ke-tooltip-inner">' +
' <span class="ke-tooltip-text">Append text</span>' +
' </span>' +
' </button></li>' +
' </ul>' +
'</div>';
return listDiv;
},
// Called after the submenu has been rendered
on: function () {
this.context.custom.textElement.focus();
}
onClick: function () {
// Get Input value
const value = this.util.createTextNode(this.context.custom.textElement.value);
// insert
this.insertNode(value);
// set range
this.setRange(value, value.length, value, value.length);
// submenu off
this.submenuOff();
// focus
this.focus();
}
}
import kothing-editor from '../src/editor'
import custom_plugin_submenu from '../test/custom_plugin_submenu'
kothing-editor.create(document.getElementById('ex_submenu'), {
plugins: [custom_plugin_submenu],
buttonList: [
['undo', 'redo'],
[
{
// plugin's name attribute
name: 'custom_plugin_submenu',
// name of the plugin to be recognized by the toolbar.
// It must be the same as the name attribute of the plugin
dataCommand: 'custom_plugin_submenu',
// The class name of the button to add
// ** If you want to enable it even in code view mode, add a "code-view-enabled" class **
buttonClass:'',
// HTML title attribute
title:'Custom plugin of the submenu',
// 'submenu' or 'dialog' or '' (command button)
dataDisplay:'submenu',
// HTML to be append to button
innerHTML:'<i class="ke-icon-add"></i>'
}
]
]
});